Python installation guide
I will briefly describe the first steps needed to obtain a running version on Python on your PC.
The version you need depends on what you want to do in Python. Some older projects are coded in Python version 2.xx, while nowadays 3.xx version is the most common. Since there is no backward compatibility between 2.xx and 3.xx, if you want to work with older projects you probably need the former version. Otherwise, if you are starting a project from scratch you have the freedom to choose.
Python 3.xx is the most popular version nowadays and, even if Python 4.xx will be out soon, it will be completely backward compatible. The standard package manager for Python is pip. It allows you to install and manage libraries and dependencies that aren’t distributed as part of the standard library. In general, the Python 3 installer gives you the option to install pip when installing Python on your system.
Windows
The installation procedure involves downloading the official Python .exe installer and running it on your system.
Download the Python Executable Installer here. Open your web browser and navigate to the Downloads for Windows section of the official Python website.
Select a link to download either the Windows x64 or x86 (in case of older 32-bit systems) executable installer.
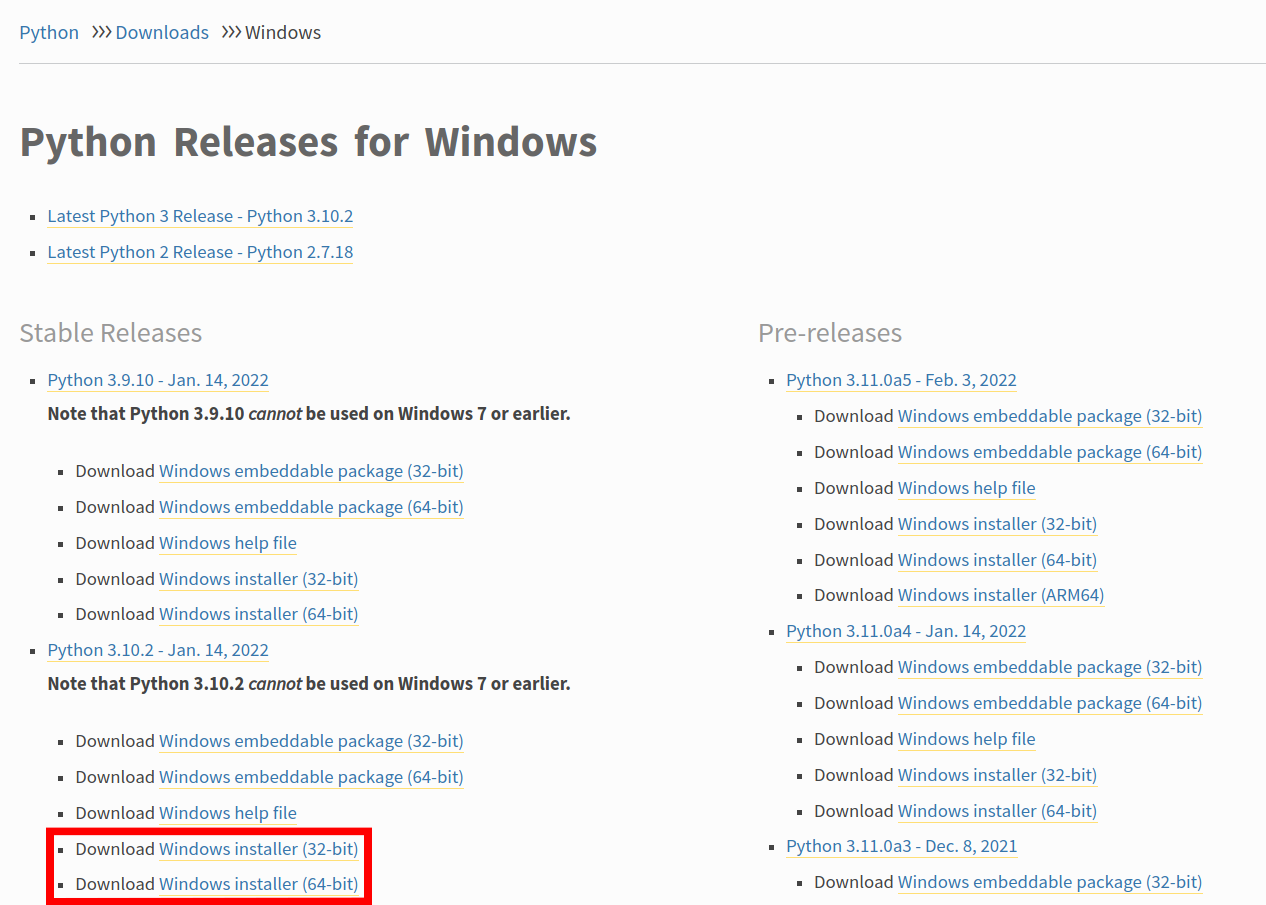
I suggest to download the latest stable release.
- Run Executable Installer and follow the instructions. In particular:
- Make sure you select the Install launcher for all users.
- Do not forget to check the “add Python to PATH” option.
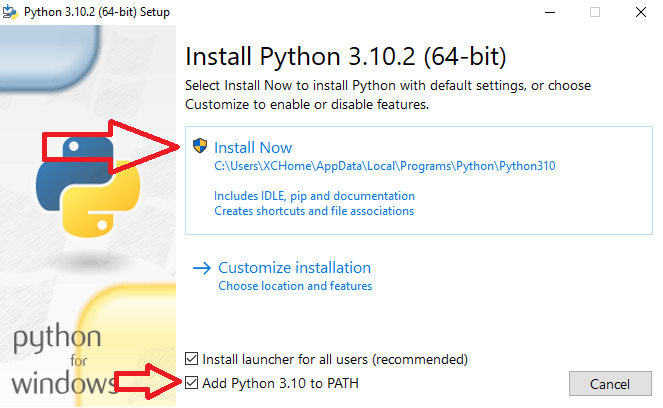
The last option will place the interpreter directly into the execution path.
The next dialog will prompt you to select whether to Disable path length limit, allowing Python to use path names longer than 260-characters.
Now both Python and pip are installed on your PC! Note that if you installed an older version of Python, it is possible that it did not come with pip preinstalled.
It is possible to verify if Python and pip are correctly installed on Windows from the Command Prompt application:
- Open the Start menu (windows icon) and type
cmd
. - Click on the Command Prompt application.
- Type
python --version
in the console and press enter. - Then enter
pip --version
in the console and press enter again.
If everything goes smoothly, you should see an output similar to the following figure.

Python and pip are correctly installed on Windows.
Mac
In general, MacOS comes with Python pre-installed, but it’s Python Version 2.xx. Then, until Apple decides to set Python 3.xx as default, you need to install it yourself.
For some of you reading this, running the python3
command from your MacOS terminal may be enough. If the software appears to not be present in your system, you can just follow the following steps:
- Open spotlight (
command + space
keys). - Type “terminal”.
- Type
brew install python3
in the console.
Homebrew will install the latest supported Python 3.xx version compatible with your OS along with pip. Otherwise, you can install pip separately following the official documentation webpage.

Python 3 interpreter is ready to accept instructions.
You now have Python 3 set up on your machine. Installation check can be performed by typing python3 --version
and pip --version
into the terminal.
Linux
To install the latest version of Python on Ubuntu Linux machines using the terminal, open a command prompt and run:
$ sudo apt update
$ sudo apt upgrade
$ sudo apt install python3
$ sudo apt install python3-pip
To see which version of Python and pip you have installed, open a command prompt and run the following commands:
$ python3 --version
$ pip --version
if everything goes right, you should see an output similar to the following:

Python and pip are correctly installed on Linux.
To launch the Python 3 interpreter it is enough to type $ python3
into the terminal.
Hello World!
Once Python is installed in your PC you are ready to program. You can compile the script directly from terminal (or command prompt in windows) by calling the built-in interpreter:
print("Hello world!")
## Hello world!
For instance, a simple segment can be plotted by using few lines of code.
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4])
plt.ylabel('some numbers')
plt.show()
For obvious reasons, the original interpreter is lightweight and (maybe excessively) simple. Alternatively, you can consider using an Integrated Development Environment (IDE). IDEs are softwares dedicated to programming, integrating several tools specifically designed for software development. The web is full of IDEs with a smart Python editor, also providing useful additional features (code navigation, code completion, refactoring, debugging and so on). Check the wiki for a not-exhaustive list of the most popular.
Further readings
- Python download main page: here you can find a list of all the versions for each supported operating system.
- Python IDEs: list of Python’s most popular Integrated Development Environments.
- Pip troubleshooting is pip installed correctly? follow this guide to solve (almost) any problem! You can also check the official pip documentation page for additional information.
- Python 2 forever: this article discusses the longevity of older Python 2.xx versions, also providing some popular projects as example.
- Differences between Python 2.xx and 3.xx: Sebastian Raschka highlights the major differences between the two versions of Python.